How to send email notifications when comments are created
Liveblocks allows you to build a commenting experience with Comments. Using our webhooks and REST API, it’s possible to send email notifications to users when they’re mentioned in comments.
What we’re building
In this guide we’ll be learning how to send comments notifications, and more specifically, we’ll be looking at how to:
- Trigger events when comments are created using the CommentCreated webhook event.
- Fetch a comment’s data using the @liveblocks/node package.
- Create notifications containing the comment’s formatted text.
- Send an email notification with Resend.
Create an endpoint in your project
When a webhook event is triggered, it can send a POST request to the back end in your project, and from within there we can send the email. In this guide, we’ll be using a Next.js route handler (API endpoint) as an example, but other frameworks work similarly.
In order to use webhooks, we’ll need to retrieve the headers
and body
from
the request. Here’s the basic endpoint we’ll be starting from:
Create this endpoint in your project, and make it available on localhost
, for
example at the following URL:
Make a note of this endpoint URL, as you’ll be using it later.
Testing webhooks locally
Running webhooks locally can be difficult, but one way to do this is to use a
tool such as localtunnel
or
ngrok
which allow you to temporarily
put your localhost server online.
If your project is running on localhost:3000
, you can run the following
command to generate a temporary URL that’s available while your localhost server
is running:
localtunnel
generates a base URL that can be placed into the Liveblocks
webhooks dashboard for quick testing. To use this, take the full address of your
webhook endpoint, and replace the domain in your localhost
address with the
generated URL.
You now have a URL that can be used in the webhooks dashboard.
Set up webhooks on the Liveblocks dashboard
To use webhooks, you need to pass your endpoint URL to the webhooks dashboard inside your Liveblocks project, and tell the webhook to trigger when a comment has been created.
Select your project
From the Liveblocks dashboard, navigate to the project you’d like to use with webhooks, or create a new project.
Go to the webhooks dashboard
Click on the “Webhooks” tab on the menu at the left.
Create an endpoint
Click the “Create endpoint…” button on the webhooks dashboard to start setting up your webhook.
Add your endpoint URL
Enter the URL of the endpoint. In a production app this will be the real endpoint, but for now enter your
localtunnel
URL from earlier.Get your webhook secret key
Click “Create endpoint” at the bottom, then find your “Webhook secret key” on the next page, and copy it.
Webhooks dashboard is set up!
Note that you can filter specifically for
commentCreated
events, but we’re ignoring this for now so we can test more easily. Let’s go back to the code.
Verify the webhook request
The @liveblocks/node
package provides
you with a function that verifies whether the current request is a real webhook
request from Liveblocks. You can set this up by setting up a
WebhookHandler
and
running verifyRequest
.
Make sure to add your “Webhook secret key” from the Liveblocks dashboard—in a real project we’d recommend using an environment variable for this.
We can then check we’re receiving the correct type of event, get the data from the webhook, and handle sending the notification inside there.
We now have the roomId
, threadId
, and commentId
of the created comment,
along with some
other information.
Get comment and thread data
The next step is to use the
Liveblocks client from
@liveblocks/node
to retrieve the entire comment’s data, along with the thread
participants. In Liveblocks Comments, a participant refers to a user that has
commented or been mentioned in a thread—we’ll be sending a notification to each
of these users.
To do this we’ll need to add our project’s secret key to the Liveblocks client,
before awaiting the following functions:
getComment
and
getThreadParticipants
.
Formatting a comment’s body
Now that we have the comment data and a list of participants, we have one more
step before sending the notifications—formatting the comment’s text, found
inside comment.body
. Let’s take a look at how it works for an example comment,
using
stringifyCommentBody
to transform comment.body
.
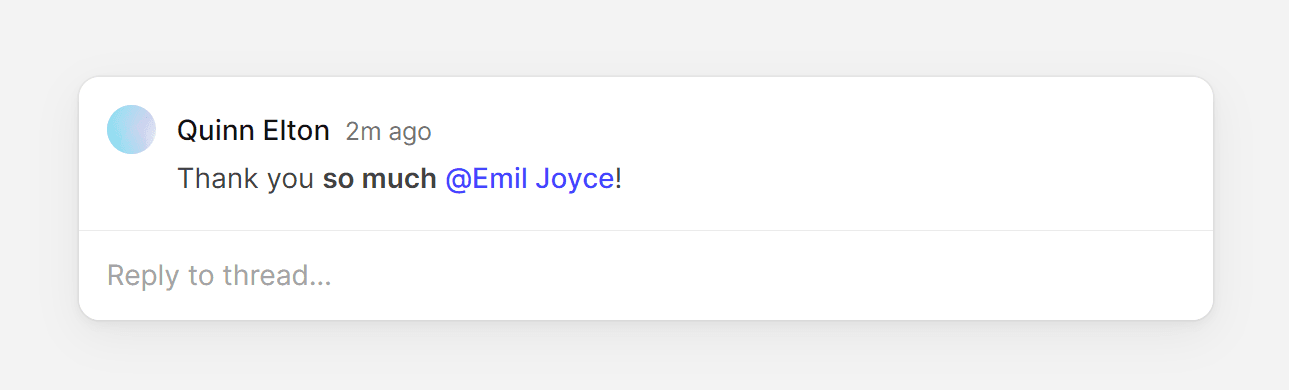
As you can see on line 6, we’re converting the body into a plain string, which means we lose the formatting. We’re also seeing the user’s ID, instead of the name—this is because we need to provide the user’s information, as the comment only stores the user’s ID.
By providing two options, we can transform the comment into HTML, keeping the formatting, and add the user information.
On line 18, you can now see that we’re creating an HTML string, and using the
mentioned user’s name. Note that you can also easily transform your comment into
Markdown, or a completely custom format, learn more under
stringifyCommentBody
.
Let’s use this HTML formatting function in our endpoint, getting user information from your database.
Send notifications
Now that the comment’s body is in our preferred format, we can send the
notifications. Earlier we retrieved participants
, a list of userIds
that
have been mentioned in the thread. You most likely have user information in your
database, which you can retrieve from these userIds
.
After getting each user’s email, simply loop through and send the formatted comment.
Sending emails with Resend
Resend is a great tool for easily sending emails, and in this code example, we’re using it to send the notifications to each user. Make sure to add your API key from the Resend dashboard before running the code.
Recap
Great, we’re successfully sending email notifications after new comments are created! In this guide we’ve learned:
- How to use webhooks and the
CommentCreatedEvent
. - How to use the
@liveblocks/node
package to get comment data and thread participants. - How to shape a comment’s body into HTML with
stringifyCommentBody
. - How to send email notifications with Resend.